Xamarin Component
TestFairy allows you to gather insights and valuable data about your Xamarin-based Android and iOS applications.
Installation
TestFairy is available for both Android and iOS. You can install TestFairy bindings for Xamarin in one of two ways:
-
Download the latest binding DLL directly from GitHub for your specific platform.
-
Install the bindings through NuGet.
You need an app token (TESTFAIRY_APP_TOKEN), which can be found on your settings page
Using the Xamarin SDK
Open AppDelegate.cs
in your solution, and override or add the following code to FinishedLaunching
.
using TestFairyLib;
...
public override bool FinishedLaunching (UIApplication app, NSDictionary options)
{
TestFairy.Begin (TESTFAIRY_APP_TOKEN);
// Rest of the method here
// ...
}
Identifying Your Users
To learn how to identify users and set session attributes using the TestFairy SDK in Xamarin, refer to the identifying users section in the SDK Documentation.
Session Attributes
For information on how to set session attributes using the TestFairy Xamarin SDK, please refer to the SDK Documentation on session attributes.
Remote Logging
To understand how to perform remote logging with the TestFairy SDK in Xamarin, refer to the remote logging section in the SDK Documentation.
Uploading dSYM
With TestFairy, symbolicating crash reports are as easy as pie. A simple Build Phase script can automatically upload the compressed .dSYM file for future symbolicaton.
To enable automatic uploads of .dSYM files, follow these steps:
-
Copy upload_dsym.sh to your project folder. Download here.
-
In Xamarin Studio, click on your project in the left sidebar, then open "Settings" and choose Options.
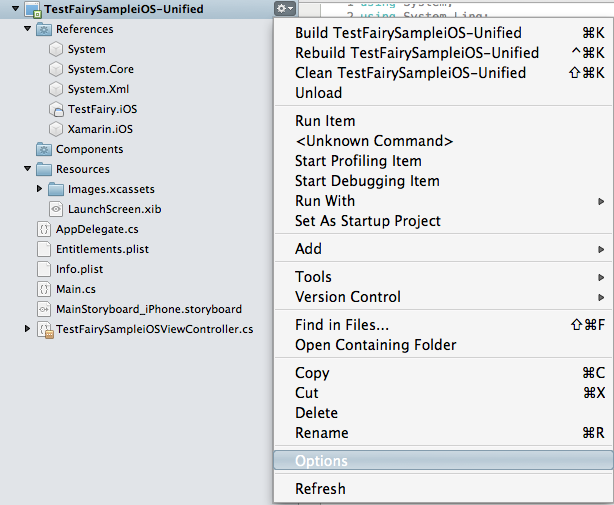
- Click on Custom Commands on the left, press Select a project operation and select After Build
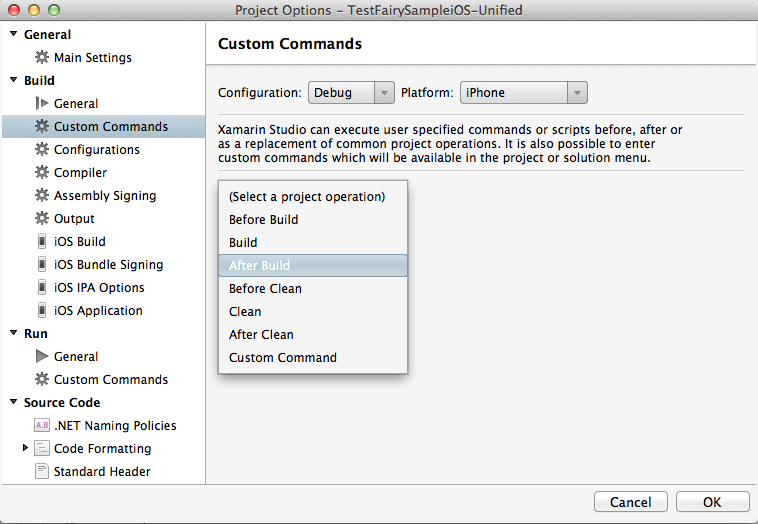
- Add the following to the command line.
sh upload-dsym.sh UPLOAD_API_KEY -p DSYM_PATH
- Replace UPLOAD_API_KEY with your upload API key; you can find it on the Settings page.
- Replace DSYM_PATH with the path of your build folder.
- Set the "Working Directory" to the path of upload-dsym.sh file
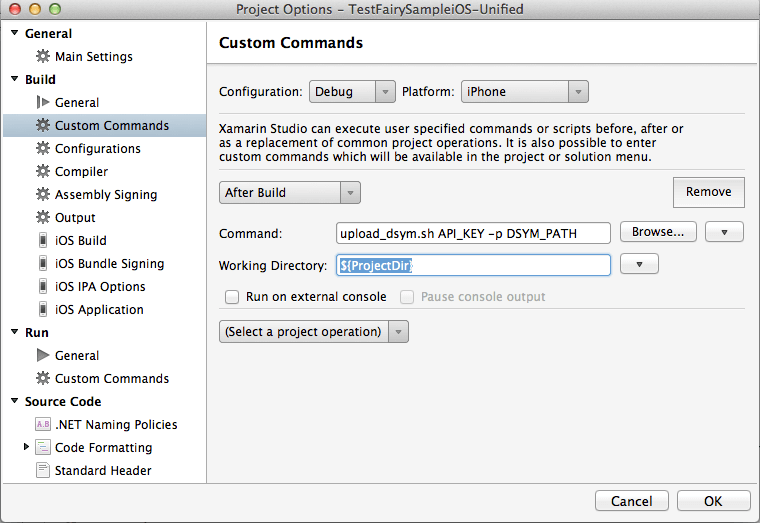
Xamarin Insights Integration
With Insights, Xamarin developers can review their app usage using the Xamarin Insights component. TestFairy fills in the gap by providing additional metrics, such as CPU usage and memory allocation and even video capture from the device. Any questions you may have as a developer will be answered in the TestFairy session reports.
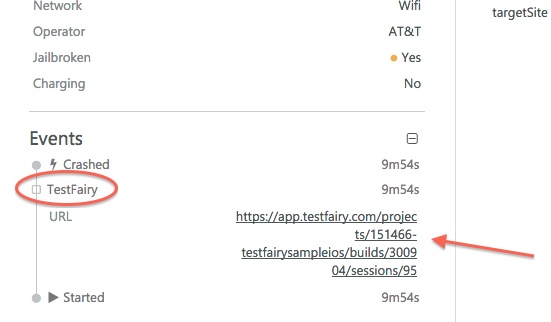
In the left sidebar of Insights, you see a link to the session recorded by TestFairy.
Integration
By adding the following code, the session recorded by TestFairy is also associated with Xamarin Insights (as seen in the screenshot above). Place this snippet right after initializing Xamarin.Insights and TestFairy.
NSNotificationCenter.DefaultCenter.AddObserver (TestFairy.SessionStartedNotification, delegate (NSNotification n) {
NSString sessionUrl = (NSString)n.UserInfo.ObjectForKey(TestFairy.SessionStartedUrlKey);
Insights.Track ("TestFairy", new Dictionary<string, string> {{ "URL", sessionUrl }});
});
Android Integration
Either in your custom Android Application class or any Activity class, call Com.TestFairy.TestFairy.Begin(<TESTFAIRY_APP_TOKEN>)
. Below is an example of invoking begin from the Main Activity.
using Com.Testfairy;
...
public class MainActivity : Activity {
protected override void OnCreate (Bundle savedInstanceState)
{
base.OnCreate (savedInstanceState);
TestFairy.Begin (this, TESTFAIRY_APP_TOKEN);
SetContentView (Resource.Layout.Main);
...
}
}
Telling TestFairy What To Record
TestFairy can record screen casts, monitor CPU consumption, memory allocations, grab logs, and enable user feedback by shaking their devices. To configure what TestFairy records, visit your Build Settings after calling Begin() at least once.
To configure how and what TestFairy records, visit your Build Settings. You see the build after calling Begin ()
at least once.
Mixing With Other Crash Handlers
TestFairy can work alongside other crash handlers without any issues.